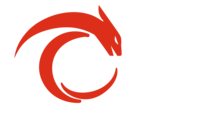 |
libdragon
|
Loading...
Searching...
No Matches
13 #define DEBUG_ADDRESS_SIZE 8*1024*1024
14 #define CHECK_EMULATOR 0
18 #define CART_64DRIVE 1
19 #define CART_EVERDRIVE 2
23 #define DATATYPE_TEXT 0x01
24 #define DATATYPE_RAWBINARY 0x02
25 #define DATATYPE_HEADER 0x03
26 #define DATATYPE_SCREENSHOT 0x04
27 #define DATATYPE_HEARTBEAT 0x05
28 #define DATATYPE_RDBPACKET 0x06
36 #define USBHEADER_GETTYPE(header) (((header) & 0xFF000000) >> 24)
37 #define USBHEADER_GETSIZE(header) (((header) & 0x00FFFFFF))
50 extern char usb_initialize(
void);
59 extern char usb_getcart(
void);
71 extern void usb_write(
int datatype,
const void* data,
int size);
81 extern unsigned long usb_poll(
void);
91 extern void usb_read(
void* buffer,
int size);
100 extern void usb_skip(
int nbytes);
109 extern void usb_rewind(
int nbytes);
117 extern void usb_purge(
void);
126 extern char usb_timedout(
void);
138 extern void usb_sendheartbeat(
void);