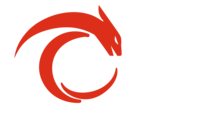 |
libdragon
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
7#ifndef __LIBDRAGON_MI_H
8#define __LIBDRAGON_MI_H
12#define MI_MODE ((volatile uint32_t*)0xA4300000)
13#define MI_VERSION ((volatile uint32_t*)0xA4300004)
14#define MI_INTERRUPT ((volatile uint32_t*)0xA4300008)
15#define MI_MASK ((volatile uint32_t*)0xA430000C)
16#define MI_BB_SECURE_EXCETPION ((volatile uint32_t*)0xA4300014)
17#define MI_BB_RANDOM ((volatile uint32_t*)0xA430002C)
18#define MI_BB_INTERRUPT ((volatile uint32_t*)0xA4300038)
19#define MI_BB_MASK ((volatile uint32_t*)0xA430003C)
21#define MI_MODE_UPPER 0x00000200
22#define MI_MODE_EBUS 0x00000100
23#define MI_MODE_REPEAT 0x00000080
24#define MI_MODE_REPEAT_COUNT 0x0000007F
26#define MI_WMODE_SET_UPPER 0x00002000
27#define MI_WMODE_CLR_UPPER 0x00001000
28#define MI_WMODE_CLR_DPINT 0x00000800
29#define MI_WMODE_SET_EBUS 0x00000400
30#define MI_WMODE_CLR_EBUS 0x00000200
31#define MI_WMODE_SET_REPEAT 0x00000100
32#define MI_WMODE_CLR_REPEAT 0x00000080
34#define MI_BB_INTERRUPT_MD_STATE 0x02000000
35#define MI_BB_INTERRUPT_BTN_STATE 0x01000000
36#define MI_BB_INTERRUPT_MD 0x00002000
37#define MI_BB_INTERRUPT_BTN 0x00001000
38#define MI_BB_INTERRUPT_USB1 0x00000800
39#define MI_BB_INTERRUPT_USB0 0x00000400
40#define MI_BB_INTERRUPT_PI_ERR 0x00000200
41#define MI_BB_INTERRUPT_IDE 0x00000100
42#define MI_BB_INTERRUPT_AES 0x00000080
43#define MI_BB_INTERRUPT_FLASH 0x00000040
44#define MI_INTERRUPT_DP 0x00000020
45#define MI_INTERRUPT_PI 0x00000010
46#define MI_INTERRUPT_VI 0x00000008
47#define MI_INTERRUPT_AI 0x00000004
48#define MI_INTERRUPT_SI 0x00000002
49#define MI_INTERRUPT_SP 0x00000001
51#define MI_BB_MASK_MD 0x2000
52#define MI_BB_MASK_BTN 0x1000
53#define MI_BB_MASK_USB1 0x0800
54#define MI_BB_MASK_USB0 0x0400
55#define MI_BB_MASK_PI_ERR 0x0200
56#define MI_BB_MASK_IDE 0x0100
57#define MI_BB_MASK_AES 0x0080
58#define MI_BB_MASK_FLASH 0x0040
59#define MI_MASK_DP 0x0020
60#define MI_MASK_PI 0x0010
61#define MI_MASK_VI 0x0008
62#define MI_MASK_AI 0x0004
63#define MI_MASK_SI 0x0002
64#define MI_MASK_SP 0x0001
66#define MI_BB_WMASK_SET_MD 0x08000000
67#define MI_BB_WMASK_CLR_MD 0x04000000
68#define MI_BB_WMASK_SET_BTN 0x02000000
69#define MI_BB_WMASK_CLR_BTN 0x01000000
70#define MI_BB_WMASK_SET_USB1 0x00800000
71#define MI_BB_WMASK_CLR_USB1 0x00400000
72#define MI_BB_WMASK_SET_USB0 0x00200000
73#define MI_BB_WMASK_CLR_USB0 0x00100000
74#define MI_BB_WMASK_SET_PI_ERR 0x00080000
75#define MI_BB_WMASK_CLR_PI_ERR 0x00040000
76#define MI_BB_WMASK_SET_IDE 0x00020000
77#define MI_BB_WMASK_CLR_IDE 0x00010000
78#define MI_BB_WMASK_SET_AES 0x00008000
79#define MI_BB_WMASK_CLR_AES 0x00004000
80#define MI_BB_WMASK_SET_FLASH 0x00002000
81#define MI_BB_WMASK_CLR_FLASH 0x00001000
82#define MI_WMASK_SET_DP 0x00000800
83#define MI_WMASK_CLR_DP 0x00000400
84#define MI_WMASK_SET_PI 0x00000200
85#define MI_WMASK_CLR_PI 0x00000100
86#define MI_WMASK_SET_VI 0x00000080
87#define MI_WMASK_CLR_VI 0x00000040
88#define MI_WMASK_SET_AI 0x00000020
89#define MI_WMASK_CLR_AI 0x00000010
90#define MI_WMASK_SET_SI 0x00000008
91#define MI_WMASK_CLR_SI 0x00000004
92#define MI_WMASK_SET_SP 0x00000002
93#define MI_WMASK_CLR_SP 0x00000001