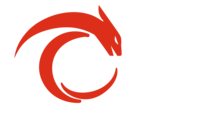 |
libdragon
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
14#ifndef __LIBDRAGON_COP1_H
15#define __LIBDRAGON_COP1_H
18#define C1_FLAG_INEXACT_OP 0x00000004
19#define C1_FLAG_UNDERFLOW 0x00000008
20#define C1_FLAG_OVERFLOW 0x00000010
21#define C1_FLAG_DIV_BY_0 0x00000020
22#define C1_FLAG_INVALID_OP 0x00000040
24#define C1_ENABLE_INEXACT_OP 0x00000080
25#define C1_ENABLE_UNDERFLOW 0x00000100
26#define C1_ENABLE_OVERFLOW 0x00000200
27#define C1_ENABLE_DIV_BY_0 0x00000400
28#define C1_ENABLE_INVALID_OP 0x00000800
29#define C1_ENABLE_MASK 0x00000F80
31#define C1_CAUSE_INEXACT_OP 0x00001000
32#define C1_CAUSE_UNDERFLOW 0x00002000
33#define C1_CAUSE_OVERFLOW 0x00004000
34#define C1_CAUSE_DIV_BY_0 0x00008000
35#define C1_CAUSE_INVALID_OP 0x00010000
36#define C1_CAUSE_NOT_IMPLEMENTED 0x00020000
37#define C1_CAUSE_MASK 0x0003F000
39#define C1_FCR31_FS (1<<24)
46#define C1_FCR31() ({ \
48 asm volatile("cfc1 %0,$f31":"=r"(x)); \
53#define C1_WRITE_FCR31(x) ({ \
54 asm volatile("ctc1 %0,$f31"::"r"(x)); \